There are infinite ways to represent a number. The four commonly associated with modern computers and digital electronics are: decimal, binary, octal, and hexadecimal.
Decimal (base 10) is the way most human beings represent numbers. Decimal is sometimes abbreviated as dec.
Decimal counting goes:
0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, and so on.
Binary (base 2) is the natural way most digital circuits represent and manipulate numbers. (Common misspellings are “bianary”, “bienary”, or “binery”.) Binary numbers are sometimes represented by preceding the value with '0b', as in 0b1011. Binary is sometimes abbreviated as bin.
Binary counting goes:
0, 1, 10, 11, 100, 101, 110, 111, 1000, 1001, 1010, 1011, 1100, 1101, 1110, 1111, 10000, 10001, and so on.
Octal (base 8) was previously a popular choice for representing digital circuit numbers in a form that is more compact than binary. Octal is sometimes abbreviated as oct.
Octal counting goes:
0, 1, 2, 3, 4, 5, 6, 7, 10, 11, 12, 13, 14, 15, 16, 17, 20, 21, and so on.
Hexadecimal (base 16) is currently the most popular choice for representing digital circuit numbers in a form that is more compact than binary. (Common misspellings are “hexdecimal”, “hexidecimal”, “hexedecimal”, or “hexodecimal”.) Hexadecimal numbers are sometimes represented by preceding the value with '0x', as in 0x1B84. Hexadecimal is sometimes abbreviated as hex.
Hexadecimal counting goes:
0, 1, 2, 3, 4, 5, 6, 7, 8, 9, A, B, C, D, E, F, 10, 11, and so on.
All four number systems are equally capable of representing any number. Furthermore, a number can be perfectly converted between the various number systems without any loss of numeric value.
At first blush, it seems like using any number system other than human-centric decimal is complicated and unnecessary. However, since the job of electrical and software engineers is to work with digital circuits, engineers require number systems that can best transfer information between the human world and the digital circuit world.
It turns out that the way in which a number is represented can make it easier for the engineer to perceive the meaning of the number as it applies to a digital circuit. In other words, the appropriate number system can actually make things less complicated.
Program
#include<stdio.h>
#include<conio.h>
#include<math.h>
int bintodec(int,int);
char digittochar(int digit)
{
if(digit<=0 && digit<=9)
return digit+48;
else
{
if(digit==10)
return'A';
else if(digit==11)
return'B';
else if(digit==12)
return'C';
else if(digit==13)
return'D';
else if(digit==14)
return'E';
else
return'F';
}
}
void rev(char a[])
{
char temp;
int i,j;
for(j=0;a[j]!='\0';j++);
j--;
for(i=0;i<j;i++,j--)
{
temp=a[i];
a[i]=a[j];
a[j]=temp;
}
}
void dectohex(int, char[],char[],int);
int main()
{
char hex[20];
int base,choice,flag,res=0;
int num;
clrscr();
while(1)
{
flag=1;
printf("Press:\n");
printf("\n\n\t1:Decimal to hexadecimal");
printf("\n\n\t2:Decimal to octal");
printf("\n\n\t3:Deciaml to binary");
printf("\n\n\t4: Binary to decimal");
printf("\n\n\t0:exit");
choice=getche();
switch(choice)
{
case'1':base=16;
break;
case'2':base=8;
break;
case'3':base=2;
break;
case'4':base=10;
break;
case'0':exit(0);
break;
default:flag=0;
}
if(flag)
{
printf("Enter the number");
scanf("%d",&num);
dectohex(num,hex,base);
if(base==16)
printf("\nHexadecimal=%s",hex);
else if(base==8)
printf("\nOctal=%s",hex);
else if(base==2)
printf("\nBinart=%s",hex);
else
printf("\nDecimmal=%d\n",bintodec(num,base));
}
}
void dectohex(int num ,char a[] ,int base )
{
int i=0,term;
while (num)
{
term=num% base;
a[i]=digittochar(term);
num=num/base;
i++;
}
a[i]='\0';
rev(a);
}
int bintodec(int num,int base)
{
int i,temp,dec=0;
for(i=0;num>0;i++)
{
temp=num%10;
dec=pow(2,i)*temp+dec;
num=num/10;
}
return();
}
}
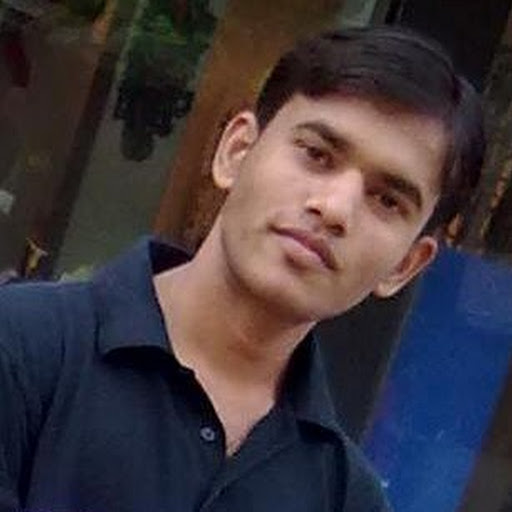
Pankaj Gaikar is a
professional blogger
from Pune, India who writes on Technology, Android,
Gadgets, social media and latest tech updates at
Punk Tech
,
Being Android
&
Shake The Tech
. Email me
HERE
0 comments :
Post a Comment