//Program for operations on queue.
#include<stdio.h>
#include<conio.h>
//Defining the structure.
struct queue
{
int data[3];
int front;
int rear;
}q;
//Initializing the queue.
void initialize()
{
q.rear=-1;
q.front=-1;
}
//Insertion of elements into queue.
void enqueue()
{
if (q.rear==2)
{
printf("\n Queue is full");
}
else
{
int ele;
printf("\nEnter the element:");
scanf("%d",&ele);
if(q.front==-1)
{
q.front++;
}
q.rear++;
q.data[q.rear]=ele;
}
}
// Deletion of elements from queue.
int dequeue()
{
int v;
if(q.front==-1||q.front>q.rear)
{
printf("\n Queue is empty:");
return(-1);
}
else
{
v=q.data[q.front];
q.front++;
return(v);
}
}
//Displaying the queue.
void display()
{
int i=q.front;
if(q.front>q.rear)
printf("\n Queue is empty:");
else
{
while(i<=q.rear)
{
printf(" %d",q.data[i]);
i++;
}
}
}
//Main Function
void main()
{
int i,data[3],ch,v;
clrscr();
initialize();
printf("\n 1.Insert 2.Delete 3.Display 4.Exit:");
do
{
printf("\n\nEnter the choice:");
scanf("%d",&ch);
switch(ch)
{
case 1: enqueue();
break;
case 2: v=dequeue();
if(v==-1)
break;
printf("Deleted element:%d",v);
break;
case 3: display();
break;
case 4: exit(0);
}
}while(ch<4);
getch();
}
//end of main
/*Output of Program:
1.Insert 2.Delete 3.Display 4.Exit:
Enter the choice:1
Enter the element:10
Enter the choice:1
Enter the element:20
Enter the choice:1
Enter the element:30
Enter the choice:1
Queue is full
Enter the choice:3
Elements in queue: 10 20 30
Enter the choice:2
Deleted element:10
Enter the choice:3
Elements in queue: 20 30
Enter the choice:1
Queue is full
Enter the choice:2
Deleted element:20
Enter the choice:2
Deleted element:30
Enter the choice:2
Queue is empty
Enter the choice:3
Queue is empty
Enter the choice:4*/
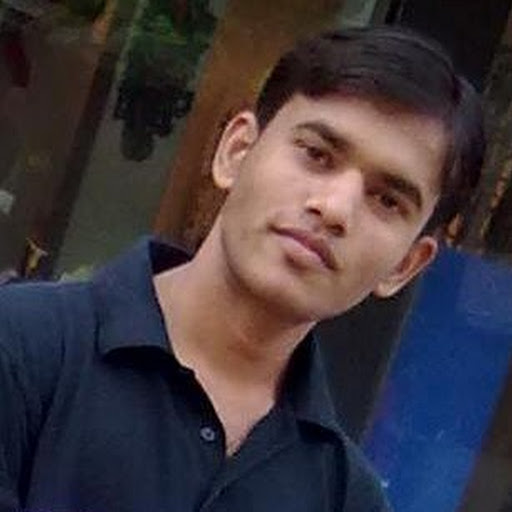
Pankaj Gaikar is a
professional blogger
from Pune, India who writes on Technology, Android,
Gadgets, social media and latest tech updates at
Punk Tech
,
Being Android
&
Shake The Tech
. Email me
HERE
0 comments :
Post a Comment