The C language provides a method to pass parameters to the main() function. This is typically accomplished by specifying arguments on the operating system command line (console).
The prototype for main() looks like:
int main(int argc, char *argv[])
{
…
}
There are two parameters passed to main(). The first parameter is the number of items on the command line (int argc). Each argument on the command line is separated by one or more spaces, and the operating system places each argument directly into its own null-terminated string. The second parameter passed to main() is an array of pointers to the character strings containing each argument (char *argv[]).
For example, at the command prompt:
test_prog 1 apple orange 4096.0
There are 5 items on the command line, so the operating system will set argc=5 . The parameter argv is a pointer to an array of pointers to strings of characters, such that:
argv[0] is a pointer to the string “test_prog”
argv[1] is a pointer to the string “1”
argv[2] is a pointer to the string “apple”
argv[3] is a pointer to the string “orange”
and
argv[4] is a pointer to the string “4096.0”
Program:
//progam for file handling with command line argument//
#include<stdio.h>
#include<conio.h>
void main(int argc,char *argv[])
{
FILE *fs,*ft;
char ch;
if(argc!=3)
{
puts("Improper number of arguments");
exit(0);
}
fs=open(argv[1],"r");
if(fs==NULL)
{
puts("can not open sourse file ");
exit(0);
}
ft=fopen(argv[2],"w");
if(fs==NULL)
{
puts("can not open target file");
fclose(fs);
exit(0);
}
while(1)
{
ch=fgetc(fs);
if(ch==EOF)
break;
else
fputc(ch,ft);
}
fclose(fs);
fclose(ft);
}
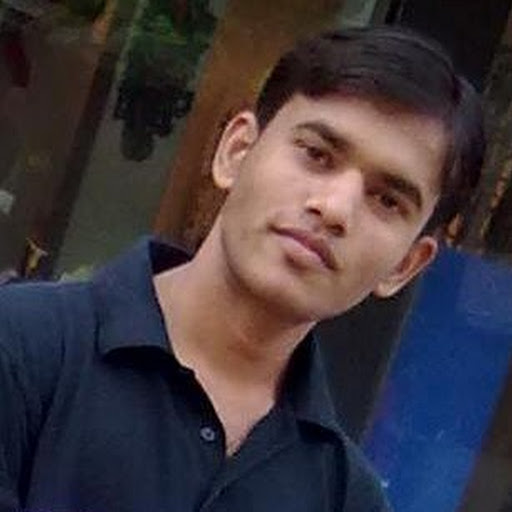
Pankaj Gaikar is a
professional blogger
from Pune, India who writes on Technology, Android,
Gadgets, social media and latest tech updates at
Punk Tech
,
Being Android
&
Shake The Tech
. Email me
HERE
0 comments :
Post a Comment