//Program to implement binary tree.
#include<conio.h>
#include<stdio.h>
#include<alloc.h>
struct tree
{
struct tree *left;
int data;
struct tree *right;
}*root;
struct tree *getnode()
{
struct tree *node,x;
node=(struct tree*)malloc(sizeof(x));
printf("enter the data for node");
scanf("%d",&node->data);
node->left=NULL;
node->right=NULL;
return (node);
}
void inorder(struct tree *root)
{
if(root->left!=NULL)
{
inorder(root->left);
}
printf("%d",root->data);
if(root->right!=NULL)
{
inorder(root->right);
}
}
void preorder(struct tree *root)
{
printf("%d",root->data);
if(root->left!=NULL)
{
preorder(root->left);
}
if(root->right!=NULL)
{
preorder(root->right);
}
}
void postorder(struct tree *root)
{
if(root->left!=NULL)
{
postorder(root->left);
}
if(root->right!=NULL)
{
postorder(root->right);
}
printf("%d",root->data);
}
void creat()
{
int flag=0;
struct tree *node,*temp;
char choice,choice1;
while(choice!='n'&&choice!='N')
{
temp=root;
flag=0;
node=getnode();
while(flag==0)
{
printf("\n\n current node=%d",temp->data);
printf("\n\n attach new node to left on right ?(l/r)");
choice1=getch();
if(root>node->data)
{
if(temp->left!=NULL)
{
temp=temp->left;
}
else
{
temp->left=node;
flag=1;
}
}
if(tolower(choice1=='r'))
{
if(temp->right!=NULL)
{
temp=temp->right;
}
else
{
temp->right=node;
flag=1;
}
}
}
printf("\n\n attach another node ? (y/n)");
choice=getch();
}
}
void search(struct tree *temp,int key)
{
temp=root;
if(temp->data==key)
{
printf("search key is found");
return;
}
if(temp->data>key)
{
if(temp->left!=NULL)
{
search(temp->left,key);
return;
}
}
if(temp->data<key)
{
if(temp->right!=NULL)
{
search(temp->right,key);
return;
}
}
printf("search key is not found");
}
void main()
{
int key;
printf("enter the value for root");
root=getnode();
creat();
printf("enter the value=");
scanf("%d",&key);
search(root,key);
printf("\n\n1.Inorder");
inorder(root);
printf("\n\n2.preorder\n\n");
preorder(root);
printf("\n\n3.postorder\n\n");
postorder(root);
getch();
}
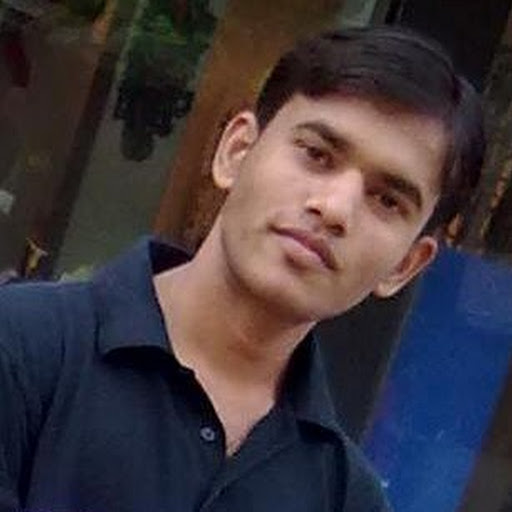
Pankaj Gaikar is a
professional blogger
from Pune, India who writes on Technology, Android,
Gadgets, social media and latest tech updates at
Punk Tech
,
Being Android
&
Shake The Tech
. Email me
HERE
0 comments :
Post a Comment