//Program for implementing Stack.
#include<stdio.h>
#include<conio.h>
#define MAX 3
//Defining structure
struct stack
{
int a[MAX];
int top;
};
struct stack s;
//Initializing stack.
void initialize()
{
s.top=-1;
}
//Push function for inserting elements onto stack.
void push(int ele)
{
s.top++;
s.a[s.top]=ele;
}
//Pop function for deleting elements from stack.
int pop()
{
int e;
if(s.top==-1)
{
return(-1);
}
else
{
e=s.a[s.top];
s.top--;
return(e);
}
}
//Displaying the stack.
void display()
{
int i=s.top;
if(s.top==-1)
{
printf("\nStack is empty");
}
else
{
printf("\nElements of stack:\n");
while(i>=0)
{
printf("%d\n",s.a[i]);
i--;
}
}
}
//Main function.
void main()
{
int ch,ele,e;
clrscr();
printf("\n 1.Push 2.Pop 3.Display 4.Exit \n");
initialize();
do
{
printf("\n\nEnter your choice:");
scanf("%d",&ch);
switch(ch)
{
case 1: if(s.top==(MAX-1))
printf("\nStack is full");
else
{
printf("\nEnter element to be inserted in the stack:");
scanf("%d",&ele);
push(ele);
}
break;
case 2: e=pop();
if(e>-1)
printf("\nDeleted element:%d",e);
else
printf("\nStack is Empty");
break;
case 3: display();
break;
case 4: exit(0);
}
}while(ch<4);
getch();
}//end of main.
/*Output of Program:
1.Push 2.Pop 3.Display 4.Exit
Enter your choice:1
Enter element to be inserted in the stack:12
Enter your choice:1
Enter element to be inserted in the stack:65
Enter your choice:3
Elements of stack:
65
12
Enter your choice:1
Enter element to be inserted in the stack:34
Enter your choice:3
Elements of stack:
34
65
12
Enter your choice:1
Stack is full
Enter your choice:2
Deleted element:34
Enter your choice:3
Elements of stack:
65
12
Enter your choice:2
Deleted element:65
Enter your choice:2
Deleted element:12
Enter your choice:2
Stack is Empty
Enter your choice:4*/
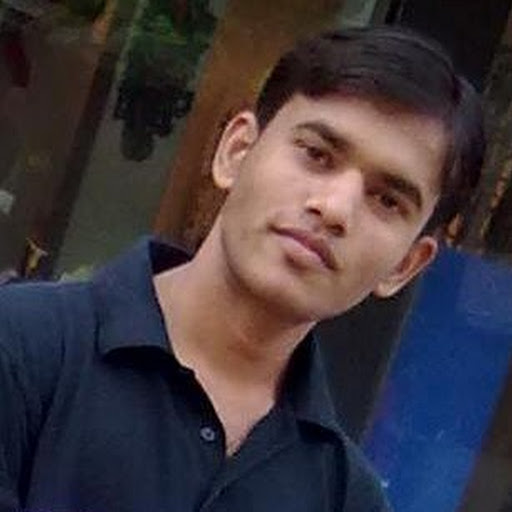
Pankaj Gaikar is a
professional blogger
from Pune, India who writes on Technology, Android,
Gadgets, social media and latest tech updates at
Punk Tech
,
Being Android
&
Shake The Tech
. Email me
HERE
0 comments :
Post a Comment