//Program for implementing Circular queue.
#include<stdio.h>
#include<conio.h>
//Defining the structure.
struct cque
{
int data[3];
int front;
int rear;
}q;
int size=3;
//Initializing the circular queue.
void initialize()
{
q.rear=-1;
q.front=-1;
}
//Enqueue Function for inserting elements into circular queue.
void cque_add()
{
int p;
p=cque_full();
if(p)
{
printf("\n Que is Full");
}
else
{ int ele;
printf("\n Enter the element:");
scanf("%d",&ele);
if(q.front==-1)
{
q.front=0;
}
q.rear=(q.rear+1)%size;
q.data[q.rear]=ele;
}
}
//Dequeue Function for deleting elements from circular queue.
int cque_delete()
{
int v,x;
x=cque_empty();
if(x)
{
printf("\n Que is Empty.");
return(-1);
}
else
{
v=q.data[q.front];
q.data[q.front]=-1;
if(q.front==q.rear)
{
q.front=-1;
q.rear=-1;
}
else
{
q.front=(q.front+1)%size;
}
return(v);
}
}
//Queue empty condition.
int cque_empty()
{
if(q.front==-1)
return(1);
else
return(0);
}
//Queue full condition.
int cque_full()
{
if(q.front==(q.rear+1)%size)
return(1);
else
return(0);
}
//Display Function for displaying circular queue.
void display()
{
int n,i=q.front;
n=cque_empty();
if(n)
printf("\n Que is Empty");
else
{
printf("\nThe elements in queue:");
do
{
if(q.data[i]!=-1)
printf(" %d ",q.data[i]);
i=(i+1)%size;
}while(i!=q.front);
}
}
//Main Function
void main()
{
int ch,v,ele,data[3];
clrscr();
initialize();
printf("1.Insert 2.Delete 3.Display 4.Exit\n");
do
{
printf("\n\n Enter the choice:");
scanf("%d",&ch);
switch(ch)
{
case 1: cque_add();
break;
case 2: v=cque_delete();
if(v!=-1)
printf("\nDeleted element:%d",v);
break;
case 3: display();
break;
case 4: exit(0);
}
}while(ch<4);
getch();
}//end of main
/*Output of Program:
1.Insert 2.Delete 3.Display 4.Exit
Enter the choice:1
Enter the element:12
Enter the choice:1
Enter the element:24
Enter the choice:1
Enter the element:45
Enter the choice:1
Que is Full
Enter the choice:3
The elements in queue: 12 24 45
Enter the choice:2
Deleted element:12
Enter the choice:3
The elements in queue: 24 45
Enter the choice:1
Enter the element:87
Enter the choice:3
The elements in queue: 24 45 87
Enter the choice:2
Deleted element:24
Enter the choice:2
Deleted element:45
Enter the choice:2
Deleted element:87
Enter the choice:2
Que is Empty.
Enter the choice:3
Que is Empty
Enter the choice:4*/
#include<stdio.h>
#include<conio.h>
//Defining the structure.
struct cque
{
int data[3];
int front;
int rear;
}q;
int size=3;
//Initializing the circular queue.
void initialize()
{
q.rear=-1;
q.front=-1;
}
//Enqueue Function for inserting elements into circular queue.
void cque_add()
{
int p;
p=cque_full();
if(p)
{
printf("\n Que is Full");
}
else
{ int ele;
printf("\n Enter the element:");
scanf("%d",&ele);
if(q.front==-1)
{
q.front=0;
}
q.rear=(q.rear+1)%size;
q.data[q.rear]=ele;
}
}
//Dequeue Function for deleting elements from circular queue.
int cque_delete()
{
int v,x;
x=cque_empty();
if(x)
{
printf("\n Que is Empty.");
return(-1);
}
else
{
v=q.data[q.front];
q.data[q.front]=-1;
if(q.front==q.rear)
{
q.front=-1;
q.rear=-1;
}
else
{
q.front=(q.front+1)%size;
}
return(v);
}
}
//Queue empty condition.
int cque_empty()
{
if(q.front==-1)
return(1);
else
return(0);
}
//Queue full condition.
int cque_full()
{
if(q.front==(q.rear+1)%size)
return(1);
else
return(0);
}
//Display Function for displaying circular queue.
void display()
{
int n,i=q.front;
n=cque_empty();
if(n)
printf("\n Que is Empty");
else
{
printf("\nThe elements in queue:");
do
{
if(q.data[i]!=-1)
printf(" %d ",q.data[i]);
i=(i+1)%size;
}while(i!=q.front);
}
}
//Main Function
void main()
{
int ch,v,ele,data[3];
clrscr();
initialize();
printf("1.Insert 2.Delete 3.Display 4.Exit\n");
do
{
printf("\n\n Enter the choice:");
scanf("%d",&ch);
switch(ch)
{
case 1: cque_add();
break;
case 2: v=cque_delete();
if(v!=-1)
printf("\nDeleted element:%d",v);
break;
case 3: display();
break;
case 4: exit(0);
}
}while(ch<4);
getch();
}//end of main
/*Output of Program:
1.Insert 2.Delete 3.Display 4.Exit
Enter the choice:1
Enter the element:12
Enter the choice:1
Enter the element:24
Enter the choice:1
Enter the element:45
Enter the choice:1
Que is Full
Enter the choice:3
The elements in queue: 12 24 45
Enter the choice:2
Deleted element:12
Enter the choice:3
The elements in queue: 24 45
Enter the choice:1
Enter the element:87
Enter the choice:3
The elements in queue: 24 45 87
Enter the choice:2
Deleted element:24
Enter the choice:2
Deleted element:45
Enter the choice:2
Deleted element:87
Enter the choice:2
Que is Empty.
Enter the choice:3
Que is Empty
Enter the choice:4*/
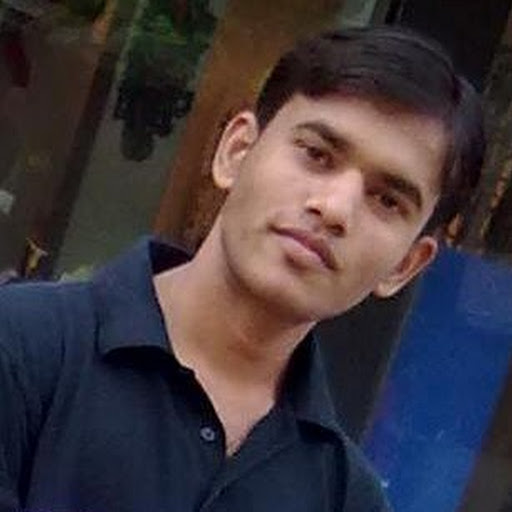
Pankaj Gaikar is a
professional blogger
from Pune, India who writes on Technology, Android,
Gadgets, social media and latest tech updates at
Punk Tech
,
Being Android
&
Shake The Tech
. Email me
HERE
0 comments :
Post a Comment